Why You Shouldn't Concatenate Strings Using "+" In Loops
Strings should not be concatenated using "+" in a loop. Learn what to do instead in this .NET tip. 💡
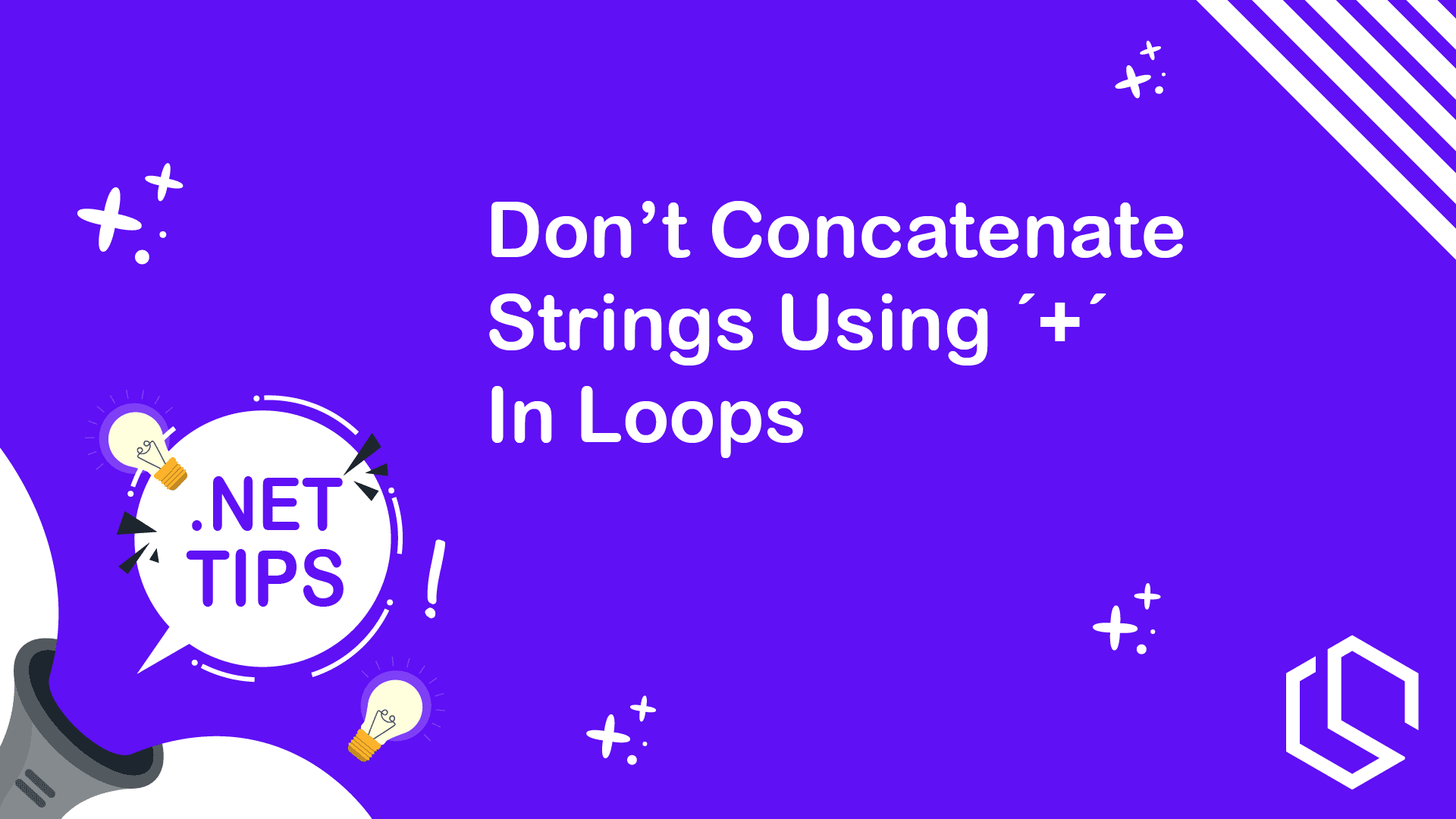
In this .NET tip I will show you why strings should not be concatenated using a +
in your loops.
I often see beginners use that operator, because it's easy and that is what they learned during the YouTube videos.. However it's not efficient and leads to bad performance. 😥
Why? 🤷♂️
Strings are immutable, which means that once a string object is created, it cannot be modified. When you concatenate strings using the +
operator in your loops, you actually creates a new string object at each iteration.
The old/previous objects are discarded of course, but it can lead to performance issues, turning your app into a snail 🐌. It can especially be a problem in large applications where you need to handle a lot of data.
The Right Way ✅
It's not rocket science and you will gain in performance 🚀 Ever heard about the StringBuilder? The StringBuilder
is designed for building strings (hence it's name 😄) in loops. It will allow you to append a strings, without having to create a new object for each iteration!
string names = string.Empty;
foreach (var name in arrayOfNames)
{
names += name;
}
var sb = new StringBuilder();
foreach(var name in arrayOfNames)
{
sb.Append(name);
}
var names = builder.ToString();
Conclusion
By using the string builder we can deal with large datasets for strings in .NET without having to sacrifice performance. It's great in conditions where you have an unknown number of strings that needs concatenation.
It will drastically reduce the memory allocations and help you improve the performance of the code in your methods.
Resources
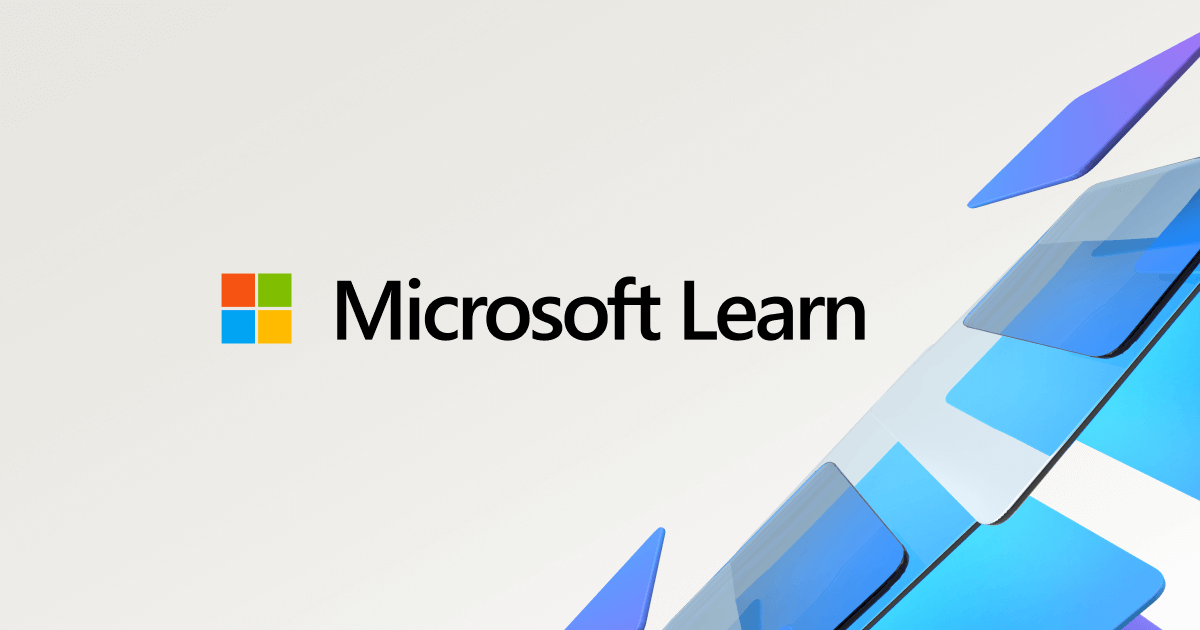
My name is Christian. I am a 28-year-old Solution Architect & Software Engineer with a passion for .NET, Cloud, and Containers. I love to share my knowledge and teach other like-minded about tech.