Use nameof to Keep Code Refactoring-Safe
Using nameof in .NET helps make code more maintainable and resilient to refactoring. It returns names as strings tied to code directly, improving readability, refactoring safety, and compile-time error checking.
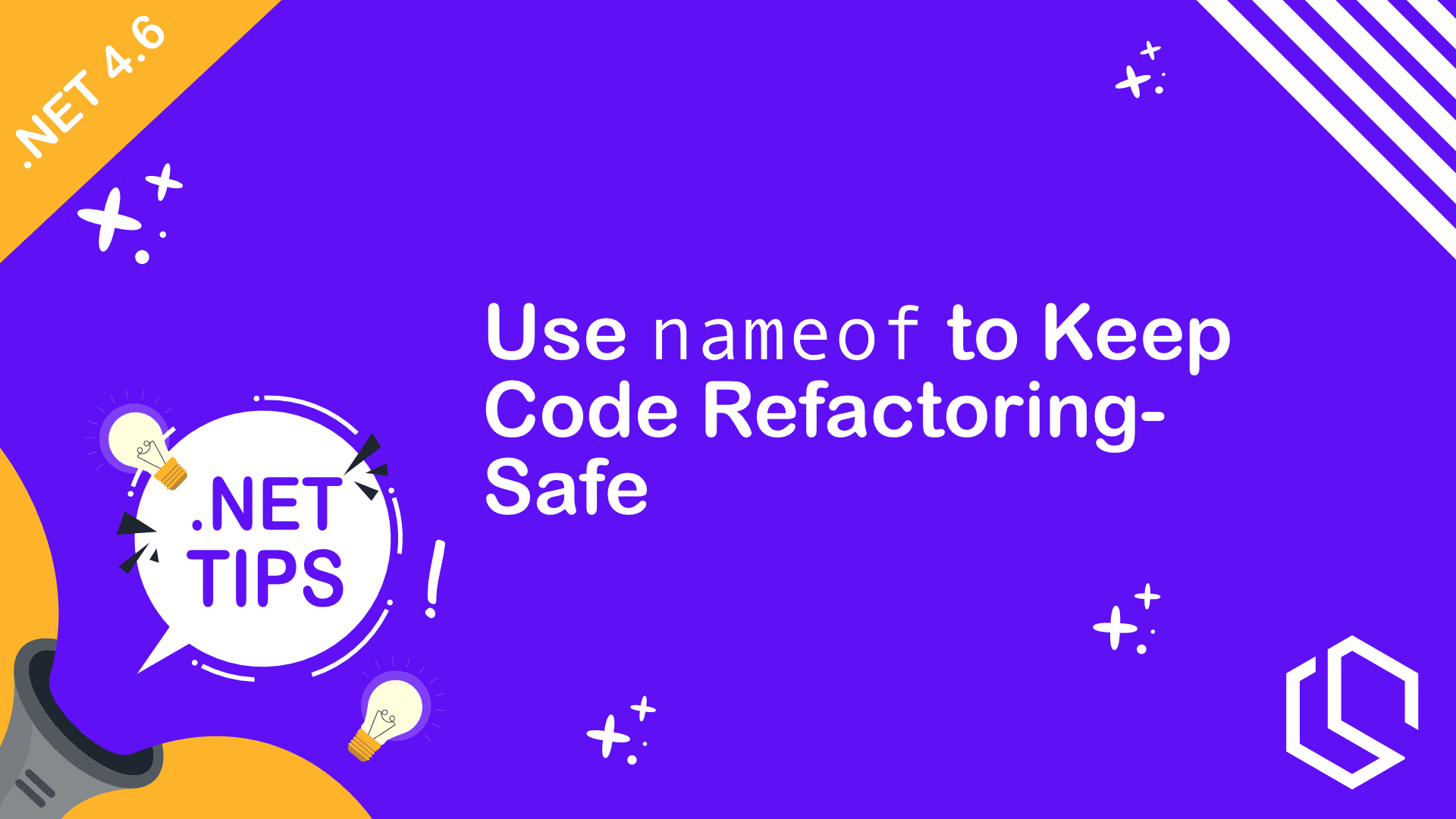
When you are writing .NET code, there is one thing I enjoy using to make my code more maintainable (and who doesn't like that?! 😅) and resilient to errors, is the use of the nameof
operator.
The nameof
operator returns the variable, type, or member as a string and nothing else. The genius thing here is that it has been tied strictly/directly to the code where it's being used, making our development much safer if we decide to refactor it.
What Is nameof?
According to Microsoft Learn, nameof
is the following:
nameof
expression produces the name of a variable, type, or member as the string constant. A nameof
expression is evaluated at compile time and has no effect at run time. - Microsoft.Why Use nameof? 🤔
So why exactly should I use the nameof
operator in my code, Christian? I am glad you asked. Here are a few reasons why I think you should do it and encourage your colleagues and other engineers to do the same.
- 👓 Readability - You can make it super clear that the value is derived from a specific variable or method, which will make your code easier to understand and as I just mentioned, refactor/maintain.
- 🧑💻 Refactoring - We have all been there, hardcoding strings... When you rename variables your methods could potentially break because it's referencing other code across your code base. When you implement nameof, your IDE will automatically update all references when you rename stuff, making it a breeze to implement changes in your code.
- ⚙️ Compiling - When you are compiling your code, you will get what I call compile-time checking. Your compiler will tell you about errors if you try to compile code where you have used a non-existing member in
nameof
. That will be another great tool in preventing compile-time errors and catching mistakes earlier.
How To Use nameof? 🧑💻
To demonstrate what I just talked about, I have created a small practical example you can reference to see how easy it is to use the nameof
operator in your own code.
public class MyClass
{
public string MyProperty { get; set; }
public void LogPropertyChange()
{
Console.WriteLine($"{nameof(MyProperty)} has changed.");
}
}
What happens above? 🤓
In the code above, nameof(MyProperty)
outputs "MyProperty"
at runtime. If you ever should rename MyProperty
, the nameof
reference updates automatically, and will prevent your compiler from complaining and errors/bugs will be equal to 0. 🐛
Resources

My name is Christian. I am a 29-year-old Solution Architect & Software Engineer with a passion for .NET, Cloud, and Containers. I love to share my knowledge and teach other like-minded about tech.