URL-Safe Base64 Encoding With .NET 9
.NET 9 introduces a new Base64Url type for URL-safe Base64 encoding, solving the issue of unsafe characters like /, +, and =.
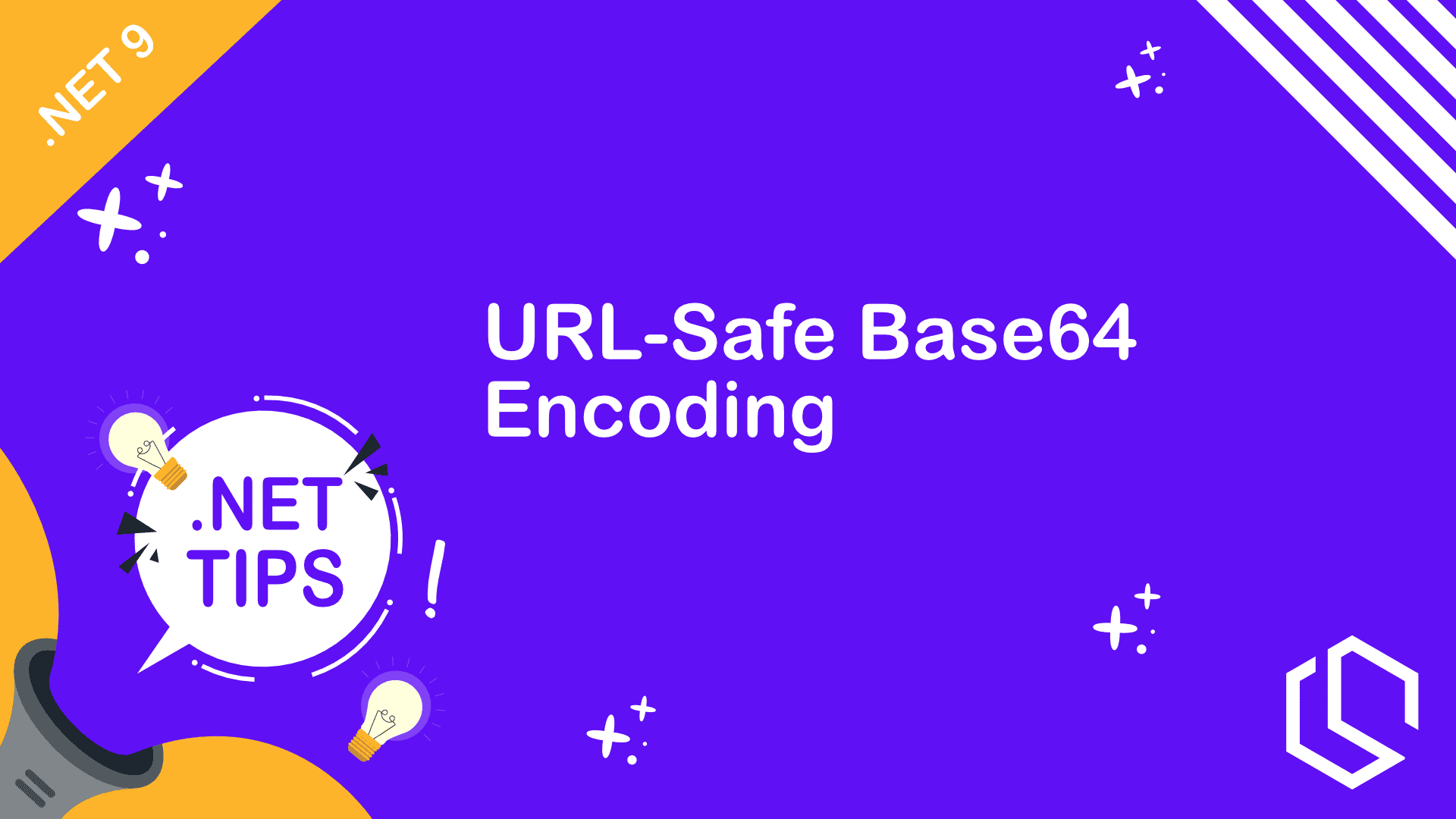
In .NET 9 we will get a new Base64Url Type. It will make it possible for developers to do URL-safe Base64 encoding on the fly.
Coming from earlier version of .NET you might think, why? We already have the Convert.ToBase64String()
method? That's correct, but that method can produce strings which contains characters such as: /
, +
,or =
. These characters are not safe for use in URL's without encoding them first, because they are used for special operations.
To fix that, the .NET team now introduces a new Base64Url.EncodeToString()
method. 👌
What Is Base64 And Why? 🤔
How To Use It 🧑💻
Here us a practical example of how to implement and use the Base64URL helper in .NET 9.
byte[] stringToEncode = Encoding.UTF8.GetBytes("Tech with Christian");
var unsafeEncoding = Convert.ToBase64String(stringToEncode);
var safeEncoding = Base64Url.EncodeToString(stringToEncode);
Console.WriteLine($"Unsafe encoding: {unsafeEncoding}");
Console.WriteLine($"Safe encoding: {safeEncoding}");
// Unsafe encoding: VGVjaCB3aXRoIENocmlzdGlhbg==
// Safe encoding: VGVjaCB3aXRoIENocmlzdGlhbg
That's how easy it is to use the new Base64Url
class with the EncodeToString
method. Just remember it's only available beginning from .NET 9.
Resources 📚
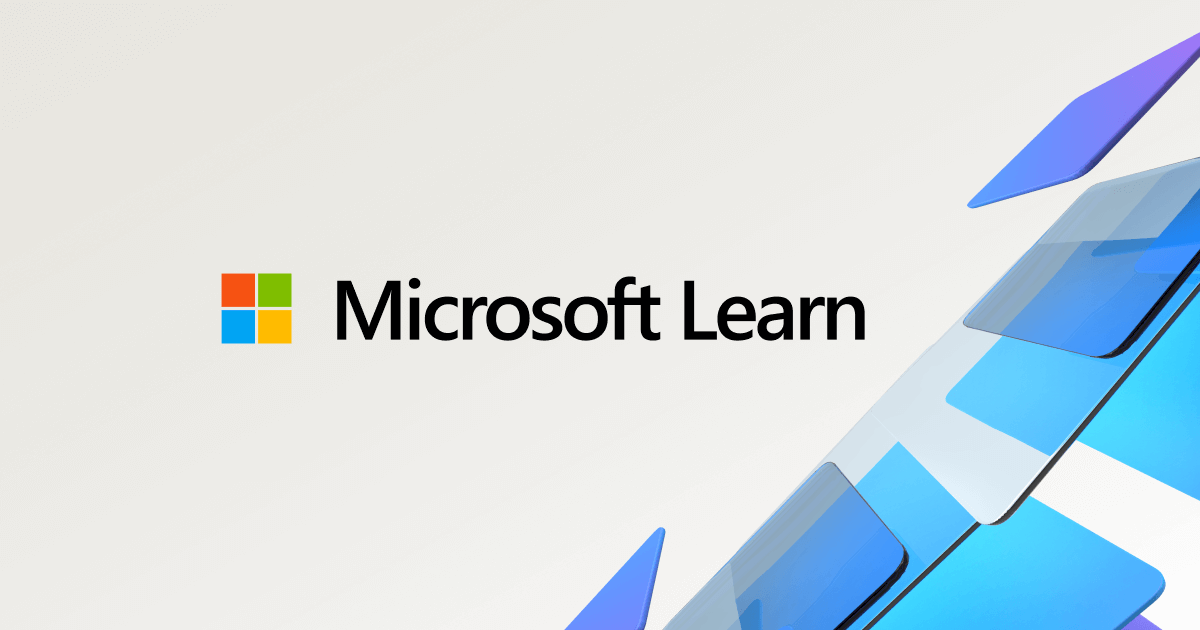
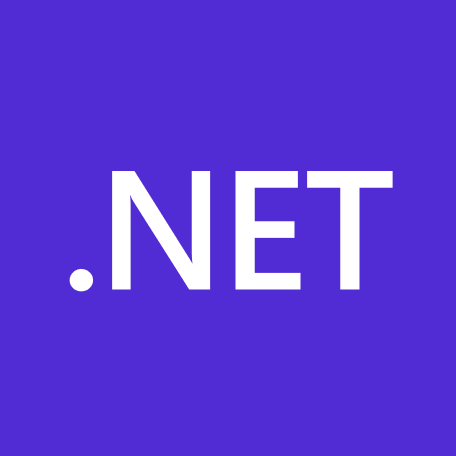
My name is Christian. I am a 28-year-old Solution Architect & Software Engineer with a passion for .NET, Cloud, and Containers. I love to share my knowledge and teach other like-minded about tech.