Replace IF-Statements With Null Conditional Operators
Ever heard of the null conditional operator (?.) in C#? It helps make your code cleaner by safely accessing members or methods that might be null, returning null instead of throwing exceptions. A handy feature from C# 6.0! ✌️
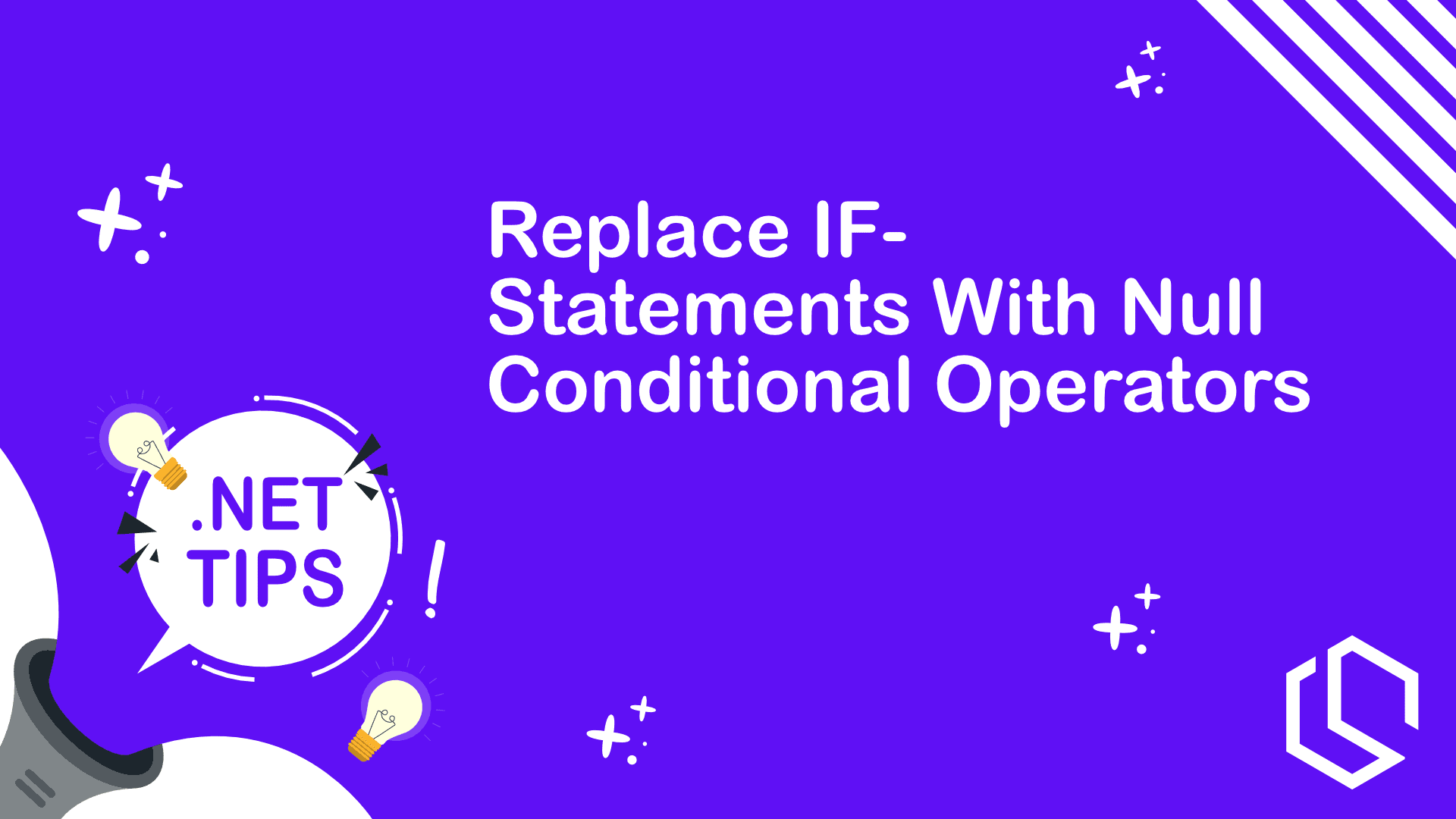
Ever heard about the null conditional operator? It is also referenced by instructors, software engineers and programmers in general as:
- Null propagation operator.
- Safe navigation operator.
It was a feature we first saw in C# 6.0 and I personally use it a lot! ✌️ It will allow you to make your code much more clean and concise, when you are working with data that might be null.
How Does It Work? 🤔
The null conditional operators is also known as a ?.
in C# (question mark with a. dot). This notation is used to access a member or invoke a method that you know might return null when invoked. If the invoked object is null, it will return null instead of throwing a new exception, genius right?! 🔥
How To Do It? 💡
Let's get to the fun part and see how we can replace an IF-statement with this null conditional operator, in practice.
if (invoice != null && invoice.Payment != null)
{
invoice.Payment.AddFee(10);
}
invoice?.Payment?.AddFee(10);
See the difference? 😅
Why Should I Do It? 🤓
I know a lot of programmers would think, yeah but I know my IF-statements and they work, why should I make the switch? Here are three reasons:
- Cleaner Code - You will reduce the need for if-statements, making your code shorter and easier to read. 🫶
- Prevents Errors - You will avoid the
NullReferenceException
by safely handling null objects. What's not to like? ✌️ - Consistent Null Checks - Standardizes null handling, improving code reliability and maintainability. Other engineers will love you! ❤️
Resources 🔗

My name is Christian. I am a 29-year-old Solution Architect & Software Engineer with a passion for .NET, Cloud, and Containers. I love to share my knowledge and teach other like-minded about tech.