Enhance String Semantics with the StringSyntax Attribute
Learn how to use the StringSyntax attribute in .NET to clarify string formats like DateTime, JSON, or Regex, enhancing readability, IDE support, and reducing errors when writing .NET or C# code ✌️
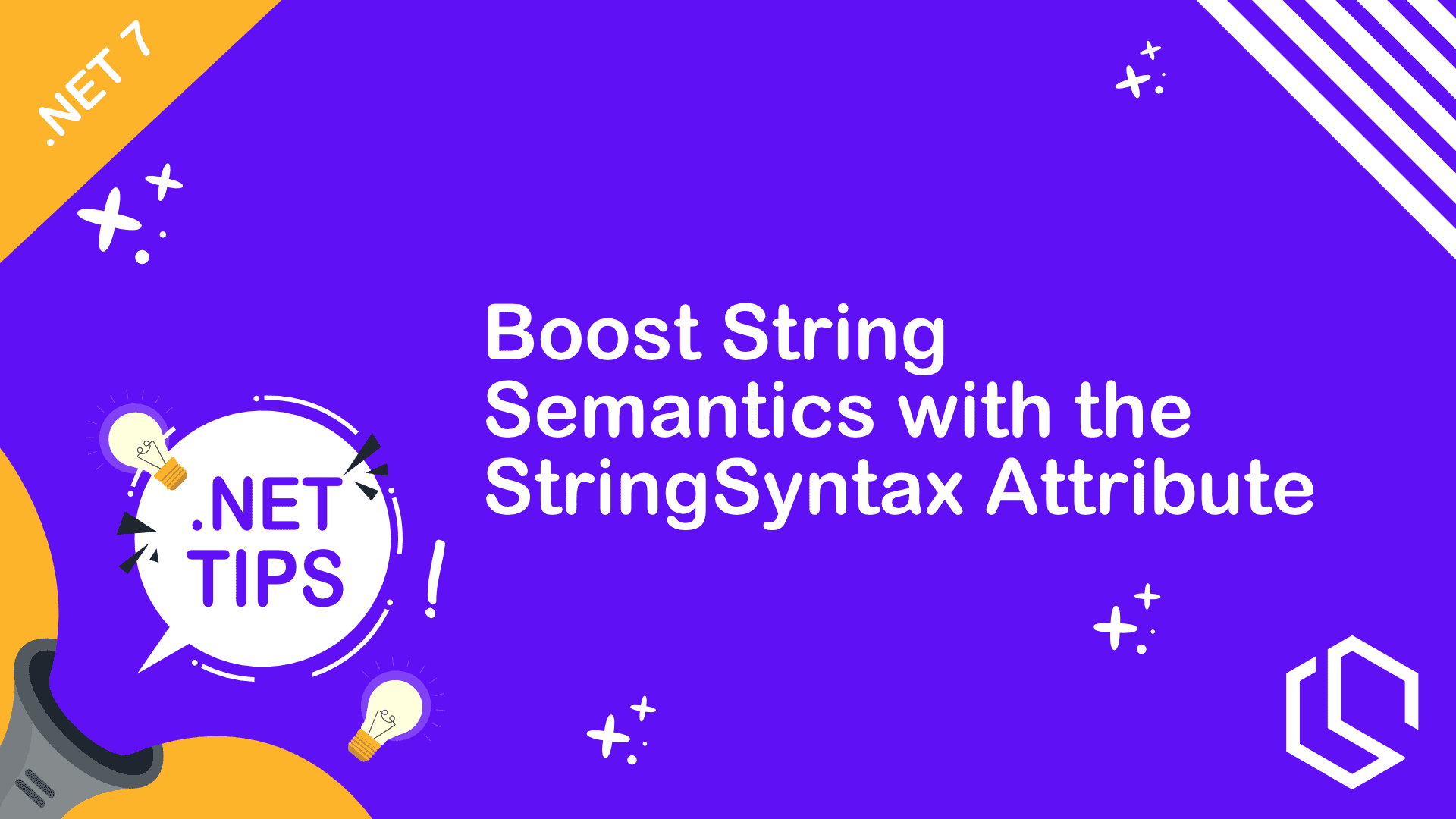
The StringSyntax
attribute was added in .NET 7, so let's just say it's a relatively new feature (at the time of writing). This new attribute allows you to specify the intended syntax or format of a string.
Having a feature like this can make our code more readable and safer. In this short .NET tip, I will show you how it works and why it is useful.
What Is StringSyntax? 🤔
The StringSyntax
attribute can only be applied to string parameters, properties, or fields in .NET. We do this to indicate to other developers (and ourselves 😅) the specific format or syntax that we expect the string to be within.
Ever heard of code analyzers? Well... by adding the StringSyntax attribute we can give them a hint about the semantic meaning of a string we are expecting. This can help with easier validation and give developers a better experience due to improved IDE support. I am talking about syntax highlighting here.
Why Use StringSyntax? 💭
You probably already have a good idea of why this is a good thing to make use of 😄 but here are my top three reasons why I think you should start using it.
- Better readability - By indicating that a string follows a specific syntax (e.g.,
Json
,Regex
, orUri
), you can make the intended purpose of the string clearer to anyone reading, refactoring, or consuming the code. ✅ - IDE support - Visual Studio and other IDEs like Rider can use the attribute to give the developer a syntax highlighting, autocompletion, and even validation on the string. What's not to like here!? 👌
- Minimize the risk of errors - By indicating a specific syntax for your string, you can avoid misinterpretation errors. Example - Imagine a method might expect a JSON-formatted string rather than plain text, and the attribute can help ensure the correct format.
How to Use StringSyntax 🧑💻
No .NET tip without a demonstration 🧑💻 Here is a quick example of how you can use StringSyntax
in your code or get an idea of how it's implemented. It's luckily pretty straightforward.
using System.Diagnostics.CodeAnalysis;
public class ExampleClass
{
public void ConfigureRegexPattern([StringSyntax(StringSyntaxAttribute.Regex)] string regexPattern)
{
// Code that uses regexPattern with the assumption it's a valid regular expression
}
public void SetJsonString([StringSyntax(StringSyntaxAttribute.Json)] string jsonString)
{
// Code that processes jsonString as JSON
}
}
Available Syntax Options ✅
The StringSyntax
attribute class provides several common syntax types, you can read more about those in the link below in my resources for this .NET tip.
Regex
- Marks the string as a regular expression pattern.Json
- Indicates the string should follow JSON formatting.DateTimeFormat
- Indicates the string should followDateTime
formatting.Uri
- Indicates aURI
format is expected.Xml
- Indicates XML format.
Use Cases ⚡️
I have tried to think about some use cases for this attribute, and here are my thoughts so far for when I would use it myself.
API Design
Helps clarify what specific string values are intended for in APIs, especially for complex formats like JSON or regular expressions.
Tooling and Analyzers
Can help static analysis tools provide warnings if the string doesn’t match the expected format.
Improved Developer Experience
Improves the development experience with IntelliSense and validation support, leading to fewer bugs and better self-documenting code.
Resources 📚
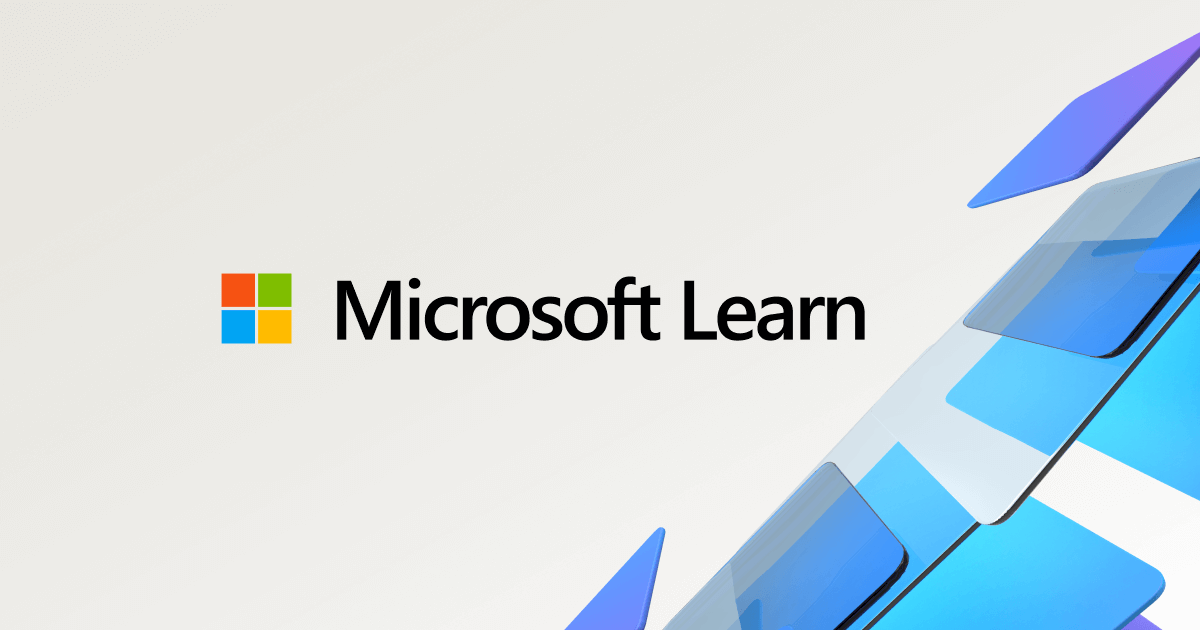
My name is Christian. I am a 29-year-old Solution Architect & Software Engineer with a passion for .NET, Cloud, and Containers. I love to share my knowledge and teach other like-minded about tech.